DUT人工智能与专家系统_HW2
1 上机要求
选择遗传算法、粒子群优化算法、蚁群算法解决旅行商问题(TSP)。要求给出最短长度和最短路径。
旅行商问题(Traveling Salesman Problem,TSP)是一种经典的组合优化问题,它涉及到在给定的一组城市之间找到最短的路径,使得每个城市都被访问一次。旅行商问题最早由爱德华·阿贝尔·哈密顿在19世纪提出,但直到20世纪才引起了人们的广泛关注。旅行商问题是一个NP难题,因此,寻找最优解的算法需要指数级的时间复杂度。
旅行商问题在许多领域都有应用,例如物流、电路板布线、DNA测序、图像处理等。在物流领域,旅行商问题可以用来优化货物的运输路线,从而降低运输成本。在电路板布线中,旅行商问题可以用来优化电路板上的连线,从而提高电路板的性能。在DNA测序中,旅行商问题可以用来优化DNA序列的测序顺序,从而提高测序的效率。在图像处理中,旅行商问题可以用来优化图像的压缩和重建,从而提高图像的质量。
为了解决旅行商问题,人们提出了许多求解算法。其中,最常用的算法包括贪心算法、动态规划算法、分支定界算法、模拟退火算法、遗传算法、蚁群算法、粒子群优化算法等。这些算法各有优缺点,可以根据具体问题的特点选择合适的算法。
2 ACO解决TSP
蚁群算法(Ant Colony Optimization,ACO)是一种基于蚂蚁行为的优化算法,它模拟了蚂蚁在寻找食物时的行为。蚁群算法的基本原理是通过信息素和启发式规则来指导每个蚂蚁的移动,从而寻找最优解。在蚁群算法中,每个蚂蚁表示一个解,它们通过不断地释放和感知信息素来寻找最优解。
对于TSP问题,蚁群算法可以被用来寻找最短的路径。在蚁群算法中,我们将问题表示为一组蚂蚁,其中每个蚂蚁表示一个解。我们使用信息素和启发式规则来指导每个蚂蚁的移动,并使用适应度函数来评估每个蚂蚁的质量。在TSP问题中,适应度函数可以是路径长度。通过不断迭代,我们可以找到最短的路径。
具体来说,蚁群算法可以通过以下步骤来解决TSP问题:
- 初始化信息素:将每条边的信息素初始化为一个较小的正数。
- 初始化蚂蚁:随机生成一组初始解,每个解表示一个蚂蚁。
- 选择下一个城市:根据信息素和启发式规则来选择下一个城市。
- 更新信息素:根据蚂蚁的路径长度来更新信息素。
- 重复步骤4,直到达到停止条件。
- 输出最优解:输出路径长度最短的蚂蚁的路径作为最优解。
需要注意的是,蚁群算法的性能取决于参数的选择,例如信息素挥发率、信息素增量、启发式规则等。因此,在使用蚁群算法解决TSP问题时,需要进行参数调整以获得最佳性能。
2.1 Python编程实现
1 |
|
2.2 输出结果
即展示前10次与后10次迭代结果。
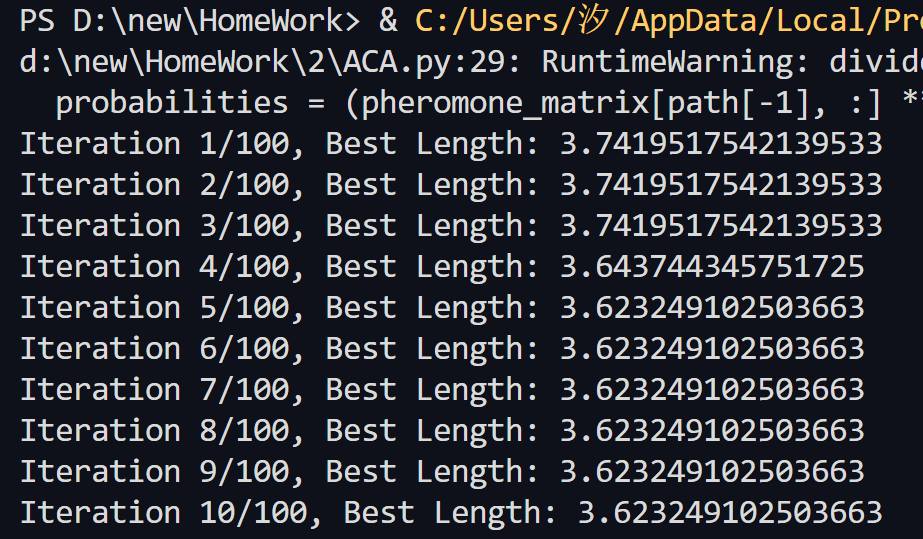
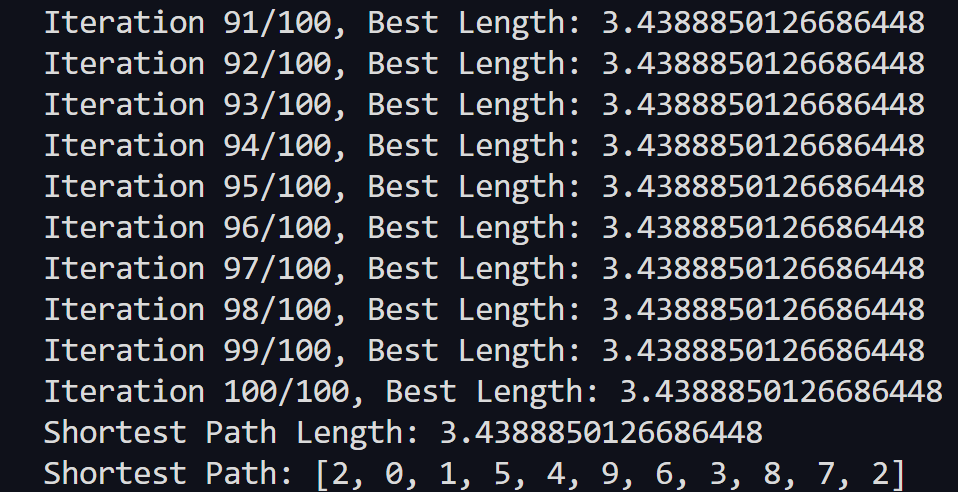
3 PSO解决TSP
粒子群优化算法(Particle Swarm Optimization,PSO)是一种基于群体智能的优化算法,它模拟了鸟群或鱼群等群体的行为。粒子群算法的基本原理是通过不断地更新每个粒子的速度和位置来寻找最优解。在粒子群算法中,每个粒子表示一个解,它们通过不断地交流信息来寻找最优解。
对于TSP问题,粒子群算法可以被用来寻找最短的路径。在粒子群算法中,我们将问题表示为一组粒子,其中每个粒子表示一个解。我们使用速度和位置更新规则来移动每个粒子,并使用适应度函数来评估每个粒子的质量。在TSP问题中,适应度函数可以是路径长度。通过不断迭代,我们可以找到最短的路径。
具体来说,粒子群算法可以通过以下步骤来解决TSP问题:
- 初始化粒子群:随机生成一组初始解,每个解表示一个粒子。
- 评估适应度:使用适应度函数来评估每个粒子的质量,适应度函数可以是路径长度。
- 更新速度和位置:使用速度和位置更新规则来移动每个粒子,以便它们可以更好地探索搜索空间。
- 更新最优解:记录全局最优解和每个粒子的最优解。
- 重复步骤4,直到达到停止条件。
- 输出最优解:输出全局最优解作为最优解。
需要注意的是,粒子群算法的性能取决于参数的选择,例如粒子数量、惯性权重、加速度系数等。因此,在使用粒子群算法解决TSP问题时,需要进行参数调整以获得最佳性能。
3.1 Python编码实现
1 |
|
3.2 输出结果
4 GA解决TSP
遗传算法是一种基于自然选择和遗传学原理的优化算法,它模拟了自然界中的进化过程。遗传算法的基本原理是通过不断地交叉和变异来生成新的解,并使用适应度函数来评估每个解的质量。在遗传算法中,解被表示为一个染色体,其中每个基因表示一个决策变量。通过不断迭代,遗传算法可以找到最优解。
对于TSP问题,遗传算法可以被用来寻找最短的路径。在遗传算法中,我们将问题表示为一个染色体,其中每个基因表示一个城市。我们使用交叉和变异操作来生成新的染色体,并使用适应度函数来评估每个染色体的质量。在TSP问题中,适应度函数可以是路径长度。通过不断迭代,我们可以找到最短的路径。
具体来说,遗传算法可以通过以下步骤来解决TSP问题:
- 初始化种群:随机生成一组初始解,每个解表示一个染色体。
- 评估适应度:使用适应度函数来评估每个染色体的质量,适应度函数可以是路径长度。
- 选择操作:使用选择操作来选择优秀的染色体,以便它们可以被用来生成下一代。
- 交叉操作:使用交叉操作来生成新的染色体,以便它们可以被用来生成下一代。
- 变异操作:使用变异操作来引入新的基因,以便它们可以被用来生成下一代。
- 重复步骤5,直到达到停止条件。
- 输出最优解:输出适应度最高的染色体作为最优解。
4.1 Python编程实现
1 |
|
4.2 输出结果
仅展示前10次迭代以及后10次迭代: